Counter
This example is the same as the one in the Getting Started guide. It does add a few details that were missing over there.
See the complete code here.
Create the store​
Our Counter
store is fairly simple, as seen below. Since we are using
annotations, we have:
- included the part file
counter.g.dart
. This is generated bybuild_runner
. - created the abstract class
CounterBase
that includes theStore
mixin - created the class
Counter
that blendsCounterBase
with the mixin_$Counter
. The mixin is defined in the generated part file.
import 'package:mobx/mobx.dart';
part 'counter.g.dart';
class Counter = CounterBase with _$Counter;
abstract class CounterBase with Store {
int value = 0;
void increment() {
value++;
}
}
The above store defines a single observable value
. The action-method
increment()
is used to increment the value
. Using actions gives you a
semantic way of invoking mutating operations on the observable state.
Connect the Widget​
Now that our model for the Store
is ready, we can focus on bringing it to life
with a Widget
. Let's create an instance of our store:
final Counter counter = Counter();
Next we create a simple StatefulWidget
that renders the count and exposes the
Increment button. To make the count automatically update on changes, we wrap
the Text
widget inside an Observer
widget. Any observable that gets used in
the builder
function of Observer
is automatically tracked. When it changes,
Observer
re-renders by calling the builder
again. This can be seen in the
highlighted code below.
class CounterExample extends StatefulWidget {
const CounterExample();
CounterExampleState createState() => CounterExampleState();
}
class CounterExampleState extends State<CounterExample> {
final Counter counter = Counter();
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(
backgroundColor: Colors.blue,
title: const Text('MobX Counter'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text(
'You have pushed the button this many times:',
),
Observer(
builder: (_) => Text(
'${counter.value}',
style: const TextStyle(fontSize: 40),
)),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: counter.increment,
tooltip: 'Increment',
child: const Icon(Icons.add),
),
);
}
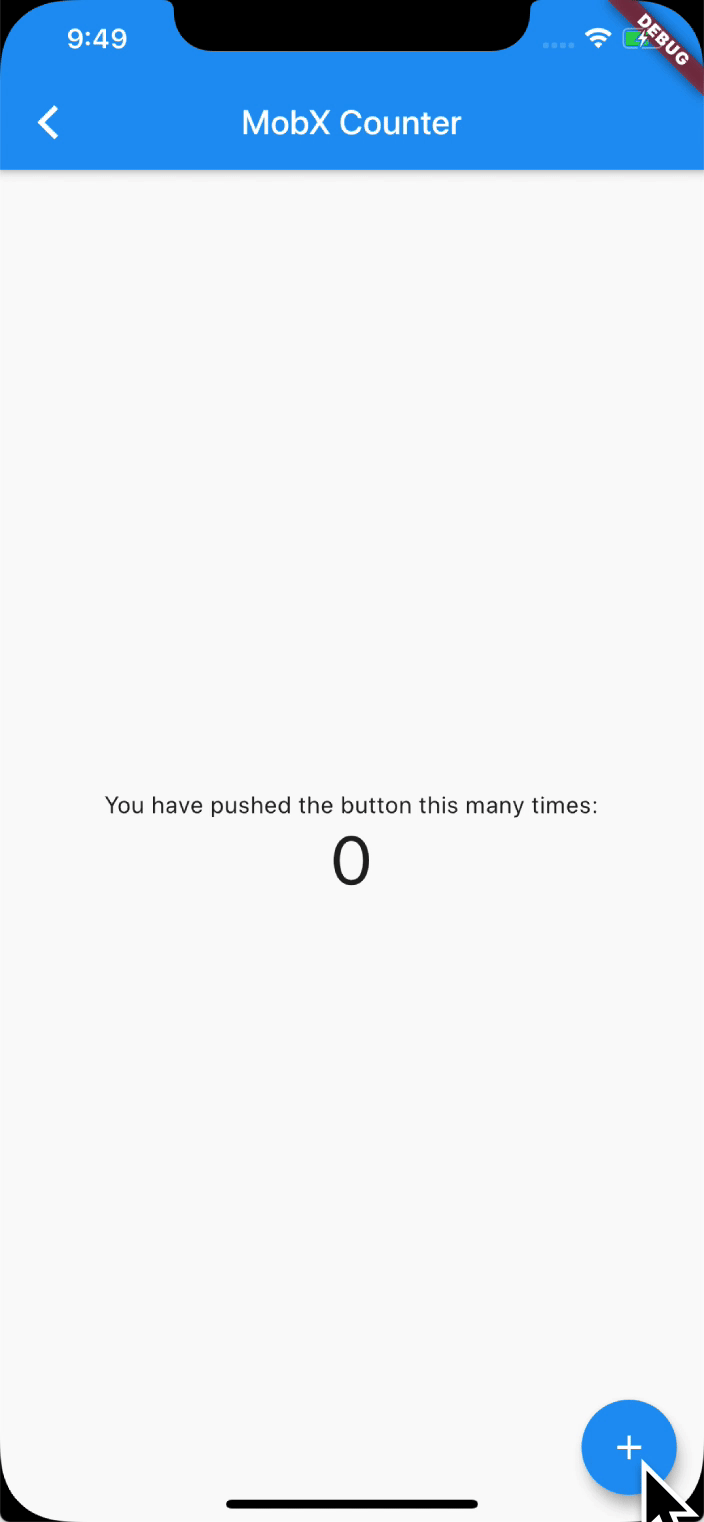
See the complete code here.