MobX for Dart and Flutter
Hassle free state-management for your Dart and Flutter apps.
Use the power of
Observables
, Actions
and Reactions
to supercharge the state in your apps.- π With official support for Null Safety
- Works on iOS, Android, Web, MacOS, Linux, Windows
- Dart 3.0 compatible
In a nutshell...
MobX is a state-management library that makes it simple to connect the reactive data of your application with the UI (or any observer). This wiring is completely automatic and feels very natural. As the application-developer, you focus purely on what reactive-data needs to be consumed without worrying about keeping the two in sync.
Let's see in code...
Classic Counter example in MobX
import 'package:flutter/material.dart';
import 'package:flutter_mobx/flutter_mobx.dart';
import 'package:mobx/mobx.dart';
class SimpleCounter {
// Step 1
final count = Observable(0);
// Step 2
void increment() {
runInAction(() => count.value++);
}
}
class CounterView extends StatefulWidget {
const CounterView({Key? key}) : super(key: key);
CounterExampleState createState() => CounterExampleState();
}
class CounterExampleState extends State<CounterView> {
final SimpleCounter counter = SimpleCounter();
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(
backgroundColor: Colors.blue,
title: const Text('MobX Counter'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text(
'You have pushed the button this many times:',
),
// Step 3
Observer(
builder: (_) => Text(
'${counter.count.value}',
style: const TextStyle(fontSize: 40),
)),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: counter.increment,
tooltip: 'Increment',
child: const Icon(Icons.add),
),
);
}
We first setup the observable state. In this case its a simple count as an integer. This forms the reactive state of our example.Step 1
Observable State
Setup the action to increment the count. When the action is executed, it will fire notifications automatically and inform all associated reactions.Step 2
Action to mutate state
Display the count using theStep 3
Reaction to observe stateObserver
. Internally, the Observer is a reaction that tracks changes to the associated observable (count). Tracking happens automatically. The simple act of reading an observable value is enough. When the count changes, it gets notified by the action and re-renders the Flutter Widget to show the updated count.
mobx_codegen
package.Tried, tested and said...
It's like BLoC, but actually works.
Remi Rousselet
Flutter enthusiast, creator of the flutter_hooks, provider packages.
We at GeekyAnts have used MobX.dart in many Flutter projects. We have chosen it as the default state-management library for the internal frameworks built for Flutter.
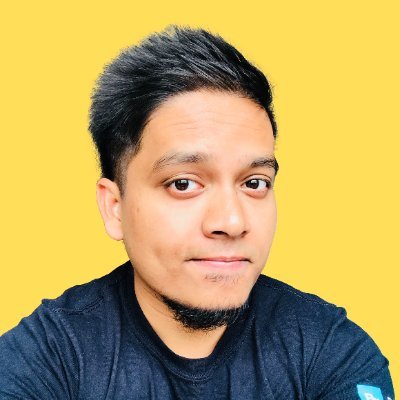
Sanket Sahu
Founder of GeekyAnts β’ Creator of BuilderXio, NativeBaseIO & VueNativeIO β’ Speaker β’ Loves tea β’ Strums guitar
If you haven't seen MobX.dart, check it out. In combination with Provider, it's PFM (Pure Flutter Magic). I use it when I build anything real. #recommended #Flutter
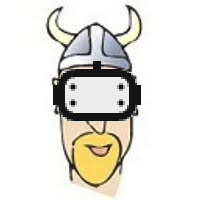
Chris Sells
Ex-Product Manager on the Flutter Development Experience. Enjoys long walks on the beach and various technologies.
MobX's concepts of Observables, Actions and Reactions make it intuitive to figure out how to model the state of an application. I would highly recommend it as a solution for managing the application's state.
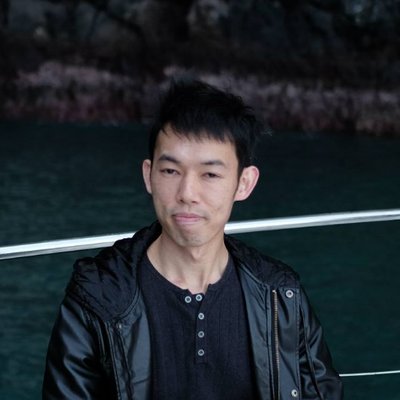
Michael Bui
Flutter Enthusiast and maintainer of flutter_local_notifications and flutter_appauth packages.
On Professional projects, MobX helps me manage hundreds of forms and thousands of fields with transparency.
When working on Generative Art, it helps me create highly configurable widgets without complexity.
MobX supports me on all of my projects.
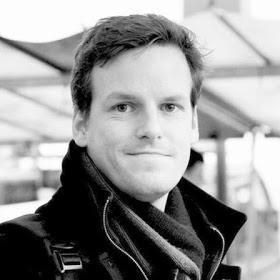
Robert Felker
#Minimalism | #flutter artist | github Awesome #flutter
MobX feels so robust and leverages the Dart language very well.
Brazil's community was previously attached to BLoC. With MobX, they have found a great replacement.
Several people here in Brazil are building Flutter apps quickly, thanks to MobX.
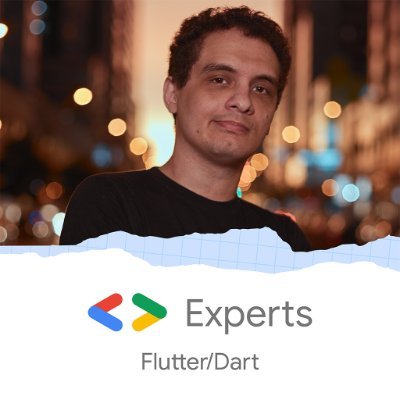
Jacob Moura
Founded the Brazilian community Flutterando. Creator of bloc_pattern, Slidy and flutter_modular packages.
This framework is just awesome. I ported my Flutter
app to it and everything feels so much simpler now (even if the porting process has not been easy).
I highly recommend it ! π
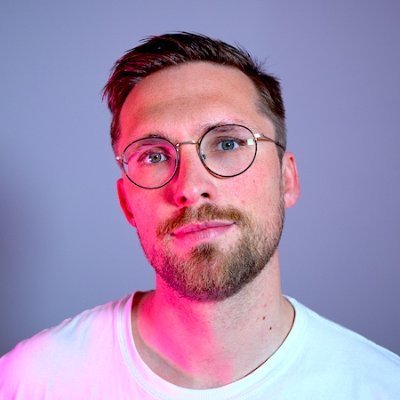
AloΓ―s Deniel
Mobile app maker in β€οΈ with Flutter & Figma & Firebase | @JintoApp co-founder | @FlutterRennes
Get Started π
Follow along with the Getting Started Guide to incorporate MobX within your Flutter project.
Go Deep π§
For a deeper coverage of MobX, do check out the MobX Quick Start Guide. Although the book uses the JavaScript version of MobX, almost all of the concepts are applicable to Dart and Flutter.
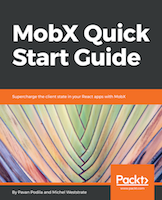